Google Analytics 4 (or GA4) has an API that provides access to data such as pageviews, traffic source, and other data points. With this API, build custom dashboards, automate reporting, and integrate with other applications.
The API response is accessed using a programming language. This guide focuses on how to access and export data using Python. We’ll cover a basic method to access data that we’re used to seeing in GA4. You can also access GA4 and import them to Google Sheets if you prefer.
But first, there’s a requirement to fetch data from the API. This requires a series of steps.
Requirement 1: Unlock the GA4 API
Let’s begin accessing the API through the Google Cloud Platform. Google Cloud Platform (or GCP) is a computing service platform from Google where you can build and deploy apps. It’s a requirement as all of the API setup is done on this platform.
Go to Google Cloud and sign-in using your Google account or Gmail on the upper-right.
On a side note, click on the screenshots to zoom in on them.
Set-Up a Google Cloud Project
First to set-up a Google Cloud Project. After signing-in, create a project.
1: Click on Select a project on the upper-left of the page.
2: Click on NEW PROJECT.
3: Give the project a name. In this example, enter ga4-project
. As for location, select No organization for now. Then click on CREATE.
4: Your new project is now created in GCP. Select the project you just created and you’ll be redirected in the workspace of GCP.
Creating the GCP project is done!
Add and Enable the API
It’s an additional (and required) step to access the API. You’re going to select and choose which API to use.
1: Inside the workspace, go to the left navigation panel and click on the following:
1 Burger icon (3 horizontal lines) > 2 APIs & Services > 3 Enabled APIs & services.
2: Inside Enabled APIs & services, click on + ENABLE APIS AND SERVICES. You’ll be redirected to the API Library
3: Inside the API Library, search for Google Analytics Data API in the search field.
4: Select Google Analytics Data API. Other APIs are here, but strictly select Google Analytics Data API.
5: Inside product details, click on ENABLE. This enables the API or get recognized by GCP.
Enabling the API is done!
Create a GCP Service Account
The next thing to do is create a service account. It’s another requirement because an email address is created. This new email is added in the GA4 property with permissions.
1: Inside the workspace, go to the left navigation panel and click on the following:
1 Burger icon (3 horizontal lines) > 2 IAM & Admin > 3 Service Accounts.
2: Inside Service Accounts, click on + CREATE SERVICE ACCOUNT.
3: Give the service account information. Follow the screenshot below for simplicity.
- Enter a name. In this example, just enter
ga4-api
. - Service account ID is auto-populated.
- Enter an optional description
- Skip Grant this service access to project and Grant users access to this service account for now.
4: Click on DONE.
This creates a [email protected]
email address. Remember this email address and take note for later.
Creating the service account done!
Download a Private Key JSON File
The next thing is to create and download a JSON file. This JSON file contains the private key and other IDs.
1: Click on the service account email address as illustrated in the screenshot above.
2: Inside Service account details, click on KEYS in the tabs.
3: Click on ADD KEY then click on Create new key.
4: In the pop-up window, select JSON. Then click on CREATE.
A JSON file is downloaded on your computer. Remember the filename as well as the file location. These things are important in the coding section later. The filename is something like ga4-project-XXXXXX-XXXXXXXXXXXX.json
Creating and downloading the private key file done!
Grant User Permissions in GA4
The next thing is to grant user permissions for the service account email address in GA4. This allows the email address to view the Google Analytics 4 property of your website.
1: Go back to Service accounts. Take note of the service account email address you created earlier. (ex. [email protected]
).
2: Go to your Google Analytics 4 property of your choice. Note: Decide on your end which GA4 property you want to use.
3: Under ADMIN, click on Property Access Management.
4: Inside Property Access Management, Click on + icon then click on Add users.
5: Inside Add roles and data restrictions, enter the service account email address and then grant Viewer access.
6: Click on Add to save it.
One more thing: you also need to remember the property ID of your Google Analytics 4 property. It’s essential in the coding section later on.
4: Under ADMIN, click on Property Settings.
5: Remember and take note of the property ID from the right side.
User permission is done!
The series of steps above can be coded in PHP, Java, Node.js, and .NET. Just so happens this guide is for Python.
Just to recap, you should remember the following for the coding section:
- JSON filename
- GA4 property ID
Requirement 2: Code in Python
You may use other programming languages. For this guide, it’s Python. I recommend using Jupyter notebook. But you may use any text editor like Visual Studio Code or Atom if you prefer.
Import Libraries
import os
import pandas as pd
import itertools
Import these libraries to continue. Install these libraries on your machine via pip install
if these aren’t installed on your computer or laptop yet.
os
library is to configure credentials.pandas
library is for DataFrames.itertools
is for querying and formatting the raw data to spreadsheet format.
Define the Property ID, and Dates
property_id = "xxxxxxxxx"
starting_date = "8daysAgo"
ending_date = "yesterday"
Next is to assign values to the GA4 property ID and dates. Remember the GA4 property that you took note of earlier? Assign that id in the property_id
variable. As for dates, starting_date
is 8daysAgo
and ending_date
is yesterday
. This is the last 7 days as an example. You may change the date values later if you wish.
Configure Google Credentials
os.environ['GOOGLE_APPLICATION_CREDENTIALS'] = 'ga4-project-xxxxxx-xxxxxxxxxxxx.json'

Remember the key and the JSON file you downloaded earlier? Assign the value here. To correctly authenticate, assign the JSON filename to GOOGLE_APPLICATION_CREDENTIALS
.
The example code above assumes that your code file is in the same folder of the JSON file. If the JSON file is located in another folder in your computer, the assigned value is different. The value also changes if you renamed the JSON file itself.
Send a Request to GA4 API
from google.analytics.data_v1beta import BetaAnalyticsDataClient
from google.analytics.data_v1beta.types import (
DateRange,
Dimension,
Metric,
RunReportRequest,
)
client = BetaAnalyticsDataClient()
request_api = RunReportRequest(
property=f"properties/{property_id}",
dimensions=[
Dimension(name="landingPagePlusQueryString")
],
metrics=[
Metric(name="sessions")
],
date_ranges=[DateRange(start_date=starting_date, end_date=ending_date)],
)
response = client.run_report(request_api)
The main purpose of this guide: getting the raw data from the API. We’re going to run the RunReportRequest
.
The request above is to get data from landingPagePlusQueryString
dimension and sessions
metric. You don’t need to change the property_id
, starting_date
, and ending_date
since you assigned values to them earlier. The thing that needs to change is the name of the dimension or metric only.
If you want to add more dimensions or metrics, add a new line to the code and make sure the syntax like adding commas are correct. You may refer to the API Dimensions & Metrics schema of Google’s documentation to know which dimension or metric you need.
Examples:Dimension(name="sessionSource")
Metric(name="conversions")
At this point, you acquired the raw data from GA4 API. If you type in and run response
, it returns the raw data from the API. Yay!
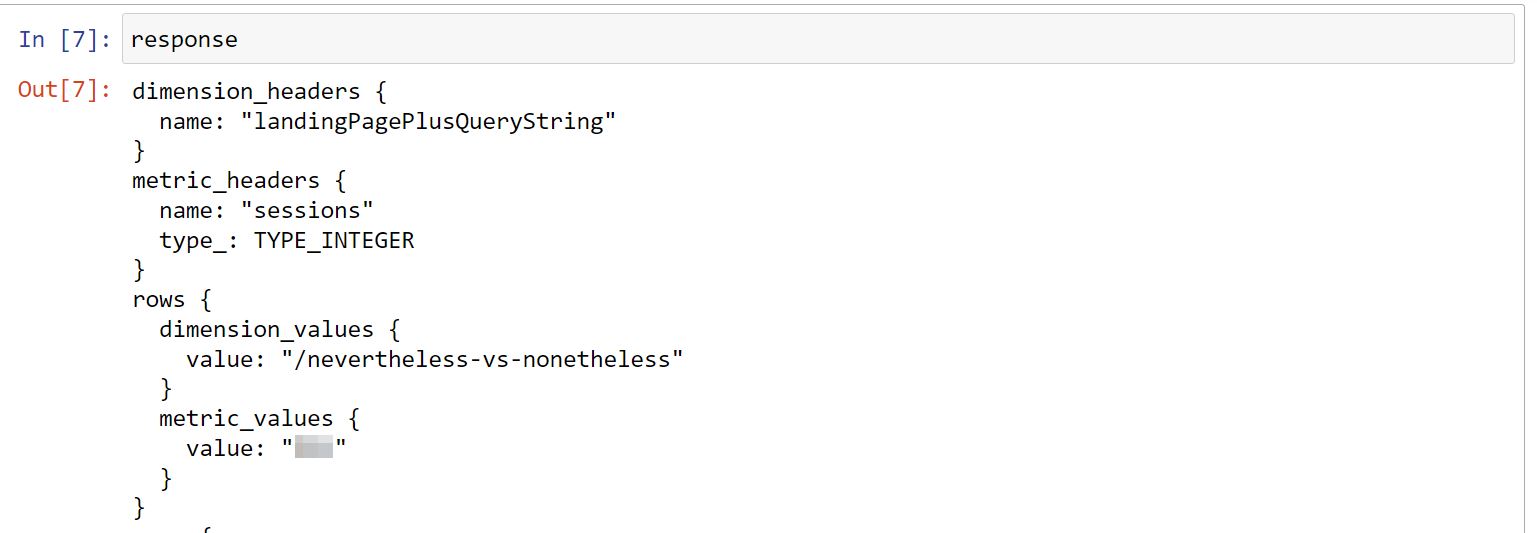
Create a DataFrame for Final Results
def query_data(api_response):
dimension_headers = [header.name for header in api_response.dimension_headers]
metric_headers = [header.name for header in api_response.metric_headers]
dimensions = []
metrics = []
for i in range(len(dimension_headers)):
dimensions.append([row.dimension_values[i].value for row in api_response.rows])
dimensions
for i in range(len(metric_headers)):
metrics.append([row.metric_values[i].value for row in api_response.rows])
headers = dimension_headers, metric_headers
headers = list(itertools.chain.from_iterable(headers))
data = dimensions, metrics
data = list(itertools.chain.from_iterable(data))
df = pd.DataFrame(data)
df = df.transpose()
df.columns = headers
return df
query_data(response)
Since you have the raw data, it’s time to format the data into a spreadsheet. The function above takes care of that. Don’t change anything in the code. Any additional dimensions or metrics still works using the function above so try testing it.
The data is now in a spreadsheet format. If you type in and run query_data(response)
, the results should now be readable.
Export To a Spreadsheet
final_data = query_data(response)
final_data.to_csv('file.csv', index=False)
To end this exercise, the code above exports the dataframe to a CSV file. The CSV file is saved in the same folder location of your code file. Done!
If you follow the instructions above, and no results were returned, it’s because of a number of reasons:
- The dimension or metric you entered do not exist. Refer to the API documentation.
- Your actual GA4 property has no data.
- The dimension and metric combination is invalid. This is Google Analytics issue rather than a code issue.
Conclusion: Make GA4 Reports from the API
Accessing the API is a good workaround if you prefer to parse through GA4 data without any restrictions from its user interface. Using the API allows any marketer to customize their workflow when needed.
Use the API in combination with other applications to create powerful integrations. The result of using the API is getting a more comprehensive understanding of Google Analytics data. With this knowledge, you can make more informed decisions about your marketing strategy.